Debugging Mule WebService Consumers
Create and debug your Mule Web Service consumer using CXF and SoapUI
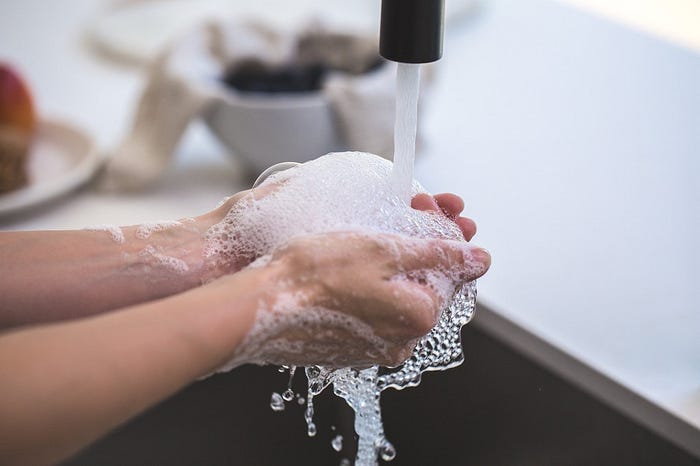
To get started download the latest Apache CXF, we’ll be using the WSDL First example as a SOAP Server to provide responses to our consumer request. In it’s simplest form, the SOAP server will run in an embedded Jetty container. If you prefer using Tomcat instead, feel free, but this guide will only cover the steps for running with Jetty.
# create a folder for CXF install
$ mkdir ~/tools
$ cd ~/tools# download CXF
$ wget https://www.apache.org/dyn/closer.lua/cxf/3.4.2/apache-cxf-3.4.2.tar.gz# verify SHA256 sum from download page
# Linux: sha256sum
# Windows:certutil -hashfile apache-cxf-3.4.2.tar.gz SHA256
# MacOs: shasum
$ shasum -a 256 apache-cxf-3.4.2.tar.gz# start the CXF server
$ cd $ ~/tools/apache-cxf-3.4.2/samples/wsdl_first
$ mvn -Pserver
The CXF example comes with a Client component for sending SOAP requests which you’re free to explore at your leisure. We’ll take a simpler approach of importing the WSDL into a new SoapUI project.

After you’ve imported the WSDL the SOAP API methods will be exposed in the navigator pane on the right.

In the XML payload,change the customer name to Smith, as it already has a canned reply in the server.

When you send your request you should get back the SOAP response which is displayed in the right hand pane. Note that the SOAP Server times out after about 5 minutes, so you may have to restart it periodically.
In Mule go ahead and create a new project which in which the SOAP Consumer flow is triggered to start when it receives an HTTP message. Your completed project will look like this:

Dataweave XML transform
%dw 2.0output application/xml
ns cus http://customerservice.example.com/
---
{
cus#getCustomersByName: {
name: "Smith"
}
}
SOAP Consumer

The Logger component simply logs the response payload. We’ll also enable a DEBUG async logger in log4j2.xml to demonstrate what you may need to do to get more information when you’re enabling more complex SOAP interactions.
<!-- Adjust log levels for more or less info -->
<AsyncLogger name="org.apache.cxf" level="DEBUG"/><!-- You may need to enable for more consumer debug -->
<!-- <AsyncLogger name="org.mule.extension.ws" level="DEBUG"/> -->
Sample debug log
DEBUG 2021-01-18 13:18:47,897 [http.listener.14 SelectorRunner] [processor: ; event: ] org.mule.service.http.impl.service.HttpMessageLogger.HTTP_Listener_config: LISTENER
GET /cxf HTTP/1.1
Host: localhost:8081
User-Agent: HTTPie/2.3.0
Accept-Encoding: gzip, deflate
Accept: */*
Connection: keep-aliveINFO 2021-01-18 13:18:48,446 [[MuleRuntime].uber.02: [soaprequest].soaprequestFlow.BLOCKING @1d188592] [processor: ; event: 9b977bb0-59b9-11eb-8419-acde48001122] org.apache.cxf.wsdl.service.factory.ReflectionServiceFactoryBean: Creating Service {http://client.internal.soap.mule.org/}ProxyService from class org.mule.soap.internal.client.CxfClientFactory$ProxyService
DEBUG 2021-01-18 13:18:48,682 [[soaprequest].wsc-dispatcher.15 SelectorRunner] [processor: ; event: ] org.mule.service.http.impl.service.HttpMessageLogger.wsc-dispatcher: REQUESTER
POST /CustomerServicePort HTTP/1.1
soapaction:
Host: localhost:9090
User-Agent: AHC/1.0
Connection: keep-alive
Accept: */*
Content-Type: text/xml; charset=UTF-8
Transfer-Encoding: chunked
e4
<soap:Envelope xmlns:soap="http://schemas.xmlsoap.org/soap/envelope/"><soap:Body><cus:getCustomersByName xmlns:cus="http://customerservice.example.com/">
<name>Smith</name>should
</cus:getCustomersByName></soap:Body></soap:Envelope>
DEBUG 2021-01-18 13:18:48,685 [[soaprequest].wsc-dispatcher.15 SelectorRunner] [processor: ; event: ] org.mule.service.http.impl.service.HttpMessageLogger.wsc-dispatcher: REQUESTERDEBUG 2021-01-18 13:18:48,689 [[soaprequest].wsc-dispatcher.15 SelectorRunner] [processor: ; event: ] org.mule.service.http.impl.service.HttpMessageLogger.wsc-dispatcher: REQUESTER
HTTP/1.1 200 OK
Date: Mon, 18 Jan 2021 18:18:48 GMT
Content-Type: text/xml;charset=utf-8
Content-Length: 664
Server: Jetty(9.4.35.v20201120)
<soap:Envelope xmlns:soap="http://schemas.xmlsoap.org/soap/envelope/"><soap:Body><ns2:getCustomersByNameResponse xmlns:ns2="http://customerservice.example.com/"><return><customerId>0</customerId><name>Smith</name><address>Pine Street 200</address><numOrders>1</numOrders><revenue>10000.0</revenue><test>1.5</test><birthDate>2009-02-01-05:00</birthDate><type>BUSINESS</type></return><return><customerId>0</customerId><name>Smith</name><address>Pine Street 200</address><numOrders>1</numOrders><revenue>10000.0</revenue><test>1.5</test><birthDate>2009-02-01-05:00</birthDate><type>BUSINESS</type></return></ns2:getCustomersByNameResponse></soap:Body></soap:Envelope>INFO 2021-01-18 13:18:48,755 [[MuleRuntime].uber.02: [soaprequest].soaprequestFlow.BLOCKING @1d188592] [processor: soaprequestFlow/processors/2; event: 9b977bb0-59b9-11eb-8419-acde48001122] org.mule.runtime.core.internal.processor.LoggerMessageProcessor: {
body:<ns2:getCustomersByNameResponse xmlns:ns2="http://customerservice.example.com/"><return><customerId>0</customerId><name>Smith</name><address>Pine Street 200</address><numOrders>1</numOrders><revenue>10000.0</revenue><test>1.5</test><birthDate>2009-02-01-05:00</birthDate><type>BUSINESS</type></return><return><customerId>0</customerId><name>Smith</name><address>Pine Street 200</address><numOrders>1</numOrders><revenue>10000.0</revenue><test>1.5</test><birthDate>2009-02-01-05:00</birthDate><type>BUSINESS</type></return></ns2:getCustomersByNameResponse>,
headers: [],
attachments: []
}DEBUG 2021-01-18 13:18:48,784 [[MuleRuntime].uber.02: [soaprequest].soaprequestFlow.BLOCKING @1d188592] [processor: ; event: 9b977bb0-59b9-11eb-8419-acde48001122] org.mule.service.http.impl.service.HttpMessageLogger.HTTP_Listener_config: LISTENER
HTTP/1.1 200
Content-Type: application/java; charset=UTF-8
Content-Length: 594
Date: Mon, 18 Jan 2021 18:18:48 GMT {
body:<ns2:getCustomersByNameResponse xmlns:ns2="http://customerservice.example.com/"><return><customerId>0</customerId><name>Smith</name><address>Pine Street 200</address><numOrders>1</numOrders><revenue>10000.0</revenue><test>1.5</test><birthDate>2009-02-01-05:00</birthDate><type>BUSINESS</type></return><return><customerId>0</customerId><name>Smith</name><address>Pine Street 200</address><numOrders>1</numOrders><revenue>10000.0</revenue><test>1.5</test><birthDate>2009-02-01-05:00</birthDate><type>BUSINESS</type></return></ns2:getCustomersByNameResponse>,
headers: [],
attachments: []
}
In the log sample above you can see the SOAP payload being sent to the Server and the Response payload from the server.
I hope this tutorial was helpful in getting you started consuming SOAP WebServices and providing insights for debugging your flow if needed.